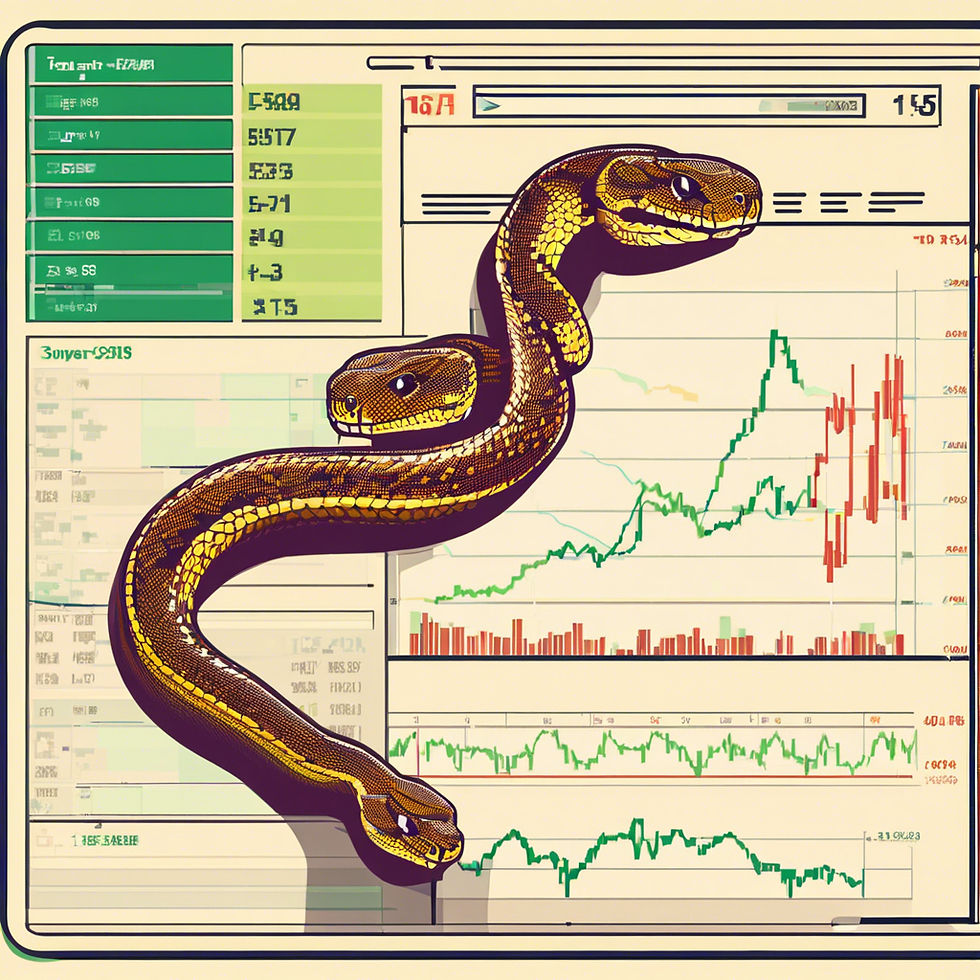
I wanted to build a top-line monitoring dashboard to track the trends of all the stocks in my portfolio. While obtaining historical data online for any stock isn't difficult, I usually download CSVs from Yahoo Finance For my previous project, the Investment Portfolio Dashboard, I downloaded the CSVs for individual stocks from Yahoo Finance and consolidated them into one CSV file. However, this process was slightly time-consuming, which led me to design a new data collection process using a few lines of Python code to leverage the YFinance API. I integrated this with a totally dynamic Streamlit interface to create a Stocks Data Downloader that allows me to download a consolidated CSV or upload my working Google Sheets (my Tableau Dashboard Datasource) in just three clicks. In this brief blog, I'll show you how to access the YFinance API using Python with just 10 lines of code.
Here's how my dynamic Streamlit User Interface looks like which we could utilise for downloading the data or updating the Google Sheets DataSource.
Installing and Importing Packages
## Installations on colab
! pip install yfinance
## Importing relevant packages
import yfinance as yf
import pandas as pd
from datetime import datetime, timedelta
Getting the Parameters
We essentially require 3 parameters to get data for our stocks. The stocks' ticker, start date and end date.
# List of tickers
tickers = ['LICI.NS', 'INFY.NS', 'HDFCBANK.NS','WIPRO.NS','TATAMOTORS.NS','BHARTIARTL.BO','TCS.NS']
# Number of years of historical data
nyears = 5
# The start and end dates
end_date = datetime.today()
start_date = end_date - timedelta(days=nyears * 365)
Please note: Here, we derive a start date based on the nyears variable to obtain the last five years of available data for each specified stock ticker. You can also use a dynamic start or end date, as I have done for the app.
The '.NS' and '.BO' suffixes used here as part of the stock tickers indicate that these stocks are listed on the National Stock Exchange (NSE) or Bombay Stock Exchange (BSE) of India, respectively. These suffixes are required because the YFinance API retrieves data from Yahoo Finance, and the stock tickers must match those on the Yahoo Finance website. To find the correct ticker symbol, you can do a quick Google search with the company name and check how it appears on Yahoo Finance.
Getting the Data and Consolidating
We call the download function of the yfinance API along with the three parameters obtained above. We must make sure that date values are in the required format using the strf function of the datetime package in python.
# Download historical data
data = yf.download(tickers, start=start_date.strftime('%Y-%m-%d'), end=end_date.strftime('%Y-%m-%d'))
Then, we extract just all the closing price columns along with the dates and stock ticker index.
# Extract just the 'Close' prices
all_closing_prices = data['Close']
We currently have the data in wide format, but we need to transform it into a long format to facilitate further analysis. This transformation can be achieved using the melt function from the Pandas package.
# Transform data to long format
final_data = all_closing_prices.reset_index().melt(id_vars='Date', var_name='Ticker', value_name='Close')
Now that we have the final data, we can export it as a CSV file, or alternatively, utilize Google APIs with an enabled service account to automatically update our Google Sheets. I've included a code snippet with the necessary prerequisites for achieving this in the additional section of the Google Colaboratory Notebook.
I hope you all will find this API and blog useful! If you have any feedback or questions, please don't hesitate to reach out. I'll be more than happy to hear from everyone.
Signing Off,
Yash
Did you find this article helpful?
Yes
No
ความคิดเห็น